The InstantMessagingMcuSessionclass represents the Instant Messaging specific implementation of the media-agnostic McuSession. The class encapsulates operations and events relevant to the Instant Messaging Multipoint Control Unit (MCU).
InstantMessagingMcuSession State
Transitions
The InstantMessagingMcuSessionstate transitions are shown in the following illustration.
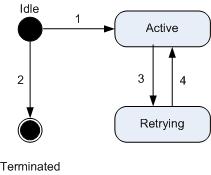
- The transition from
Idleto
Activeoccurs after
ConferenceSessionhas joined a conference. An
InstantMessagingMcuSessioninstance can become active either
before or after the callback passed to
BeginJoinis called, depending on when the MCU is activated
on the server.
- The transition from
Idleto
Terminatedoccurs when the parent
ConferenceSessionobject joins a conference that does not
support the same MCU type (for example, when an attempt is made to
join an IM-only conference to an
InstantMessagingMcuSessioninstance).
- The transition from
Activeto
Retryingoccurs when the Focus detects that the MCU is
failing over. In this case, Unified Communications Managed API 2.0
Core SDK terminates the call with that MCU, provided that the call
already exists. This transition also occurs when the
ConferenceSessionobject reconnects to the Focus. Because the
MCU is not known to be failing over, the call is not terminated in
this case.
- The transition from
Retryingto
Activeoccurs when the failover process is complete, or when
the
ConferenceSessionhas reconnected to the Focus and the MCU is
active. It is possible for the
ConferenceSessionto reconnect to the Focus, but the
InstantMessagingMcuSessionstate does not change to
Activeif the MCU has not been activated on the server.
InstantMessagingMcuSession
Constructors
The InstantMessagingMcuSessionclass has no public constructors.
InstantMessagingMcuSession
Properties
The following are the public properties on the InstantMessagingMcuSessionclass.
![]() |
|
---|---|
// Gets the media types supported by the MCU. public override IEnumerable<string> SupportedMediaTypes {get;} |
InstantMessagingMcuSession
Methods
The following are the public methods on the InstantMessagingMcuSessionclass.
![]() |
|
---|---|
// Requests that the MCU initiate a call to the entity. public IAsyncResult BeginDialOut( string destinationUri, AsyncCallback userCallback, object state); // Waits for the pending operation to complete. public new ParticipantCommandResponse EndDialOut( IAsyncResult result); // Gets the properties of the participant in this MCU. public bool TryGetParticipantEndpointProperties( ParticipantEndpoint endpoint, out InstantMessagingMcuParticipantEndpointProperties properties); // Processes added or removed participant endpoints to the MCU. protected override void HandleParticipantEndpointAttendanceChanged( Collection<KeyValuePair<ParticipantEndpoint, McuParticipantEndpointProperties>> addedEndpoints, Collection<KeyValuePair<ParticipantEndpoint, McuParticipantEndpointProperties>> removedEndpoints); // Processes updated session properties. protected override void HandlePropertiesChanged( PropertyMergeInformation<McuSessionProperties> pmi); // Processes updated MCU participant endpoint properties. protected override void HandleParticipantEndpointPropertiesChanged( ParticipantEndpoint endpoint, PropertyMergeInformation<McuParticipantEndpointProperties> pmi); // Recycles the InstantMessagingMcuSession for reuse. protected override void ResetCore(); |
InstantMessagingMcuSession
Events
The following are the public events on the InstantMessagingMcuSessionclass.
![]() |
|
---|---|
// Raised when endpoints join or leave the MCU. public event EventHandler<ParticipantEndpointAttendanceChangedEventArgs<InstantMessagingMcuParticipantEndpointProperties>> ParticipantEndpointAttendanceChanged; // Raised when a participant property changes. public event EventHandler<ParticipantEndpointPropertiesChangedEventArgs<InstantMessagingMcuParticipantEndpointProperties>> ParticipantEndpointPropertiesChanged; |