The methods and events in the RemotePresenceclass can be used to subscribe to presence information from remote presentities.
RemotePresence State
Transitions
The RemotePresencestate transitions for the subscription state of a target are shown in the following illustration. The state values are the members of the CollaborationSubscriptionStateenumerated type.
One subscription is maintained for each target whose presence is being tracked. Subscriptions to targets connected to a given server can be batched.
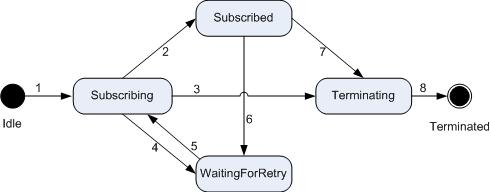
- The transition from
Idleto
Subscribingoccurs when the application calls
BeginAddTargets.
- The transition from
Subscribingto
Subscribedoccurs when the subscription to the remote
presentity succeeds.
- The transition from
Subscribingto
Terminatingoccurs when the subscription to the remote
presentity fails.
- The transition from
Subscribingto
WaitingForRetryoccurs for either of the following reasons:
- For an Office Communicator 2005 presentity, the server requests
UCMA 2.0 Core SDK to try subscribing again at a later time. At the
end of this period the Office Communicator 2005 presentity is
converted to an enhanced presence user.
- For a remote presentity that belongs to a cross pool, the
server requests UCMA 2.0 Core SDK to try subscribing to the
cross-pool server.
- For an Office Communicator 2005 presentity, the server requests
UCMA 2.0 Core SDK to try subscribing again at a later time. At the
end of this period the Office Communicator 2005 presentity is
converted to an enhanced presence user.
- The transition from
WaitingForRetryto
Subscribingoccurs within 10 seconds, when the attempt to
subscribe is made again for the reasons given in step 4.
- The transition from
Subscribedto
WaitingForRetryoccurs when the server requests UCMA 2.0 Core
SDK to close this subscription and create a new subscription. This
action can happen at any time.
- The transition from
Subscribingto
Terminatingoccurs when
BeginRemoveTargetor
BeginRemoveAllTargetsis called.
BeginRemoveTargetdoes not show this state change if the
remote presentity belongs to the home batch subscription, although
internally the remote presentity’s subscription is cancelled.
- The transition from
Terminatingto
Terminatedoccurs whether or not the subscription is
successfully ended.
RemotePresence
Constructors
The RemotePresenceclass has no public constructors.
RemotePresence Properties
There are no public properties on the RemotePresenceclass.
RemotePresence Methods
The following are the public methods on the RemotePresenceclass.
![]() |
|
---|---|
// Adds the target in the subscription dispatcher and starts the subscription for the given target. public IAsyncResult BeginAddTargets(ICollection< RemotePresentitySubscriptionTarget> targetsToAdd, AsyncCallback userCallback, object state); // Completes the asynchronous operation started by BeginAddTarget. public void EndAddTargets(IAsyncResult result); // Begins an asynchronous operation to unsubscribe to a target. public IAsyncResult BeginRemoveTarget(string uri, AsyncCallback userCallback, object state); // Completes the asynchronous operation started by BeginRemoveTarget. public void EndRemoveTarget(IAsyncResult result); // Invokes the refresh operation on all active subscriptions, client should expect to receive notifications for targets // in this batch, for which subscriptions are active. public IAsyncResult BeginRefresh(AsyncCallback userCallback, object state); // Completes the asynchronous operation started by BeginRefresh. public void EndRefresh(IAsyncResult result); // Invokes Terminate on all existing subscriptions that are not already terminating. public IAsyncResult BeginRemoveAllTargets(AsyncCallback userCallback, object state); // Completes the asynchronous operation started by BeginRemoveAllTargets. public void EndRemoveAllTargets(IAsyncResult result) // Returns the current subscription state for a given target. public CollaborationSubscriptionState GetCurrentSubscriptionState(string uri) // Begins a presence query request for a given list of targets for the given set of presence categories. // A presence query internally can involve multiple query requests to different single and pool targets. // If the user specified a query result handler, then results will be notified on the given event handler // as soon as they are available. // Calling EndPresenceQuery will return all results for the query. public IAsyncResult BeginPresenceQuery( IEnumerable<string> targets, string[] categories, EventHandler<RemotePresenceNotificationEventArgs> queryResultHandler, AsyncCallback userCallback, object state); // Ends the asynchronous operation initiated by BeginPresenceQuery. public IEnumerable<RemotePresentityNotificationData> EndPresenceQuery(IAsyncResult result); |
RemotePresence Events
The following are the public methods on the RemotePresenceclass.
![]() |
|
---|---|
// Raised when the presence state changes for any target on the subscription. public event EventHandler<RemotePresenceNotificationEventArgs> PresenceNotificationReceived; // Raised when the subscription state changes for any target. public event EventHandler<RemotePresenceSubscriptionStateChangedEventArgs> PresenceSubscriptionStateChanged; |