LocalEndpointis an abstract class that serves as the base class for the UserEndpointand ApplicationEndpointclasses. Although it would be possible to create a class derived from LocalEndpoint, developers should not attempt to do so, but should instead use the UserEndpointand ApplicationEndpointclasses.
LocalEndpoint State
Transitions
The state transitions for both endpoint types, ApplicationEndpointand UserEndpoint, are shown in the following illustrations.

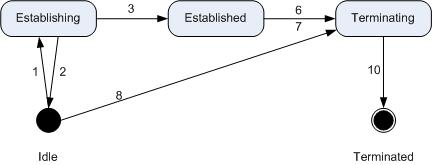
- The transition from
Idleto
Establishingoccurs when
BeginEstablishis called.
- The transition from
Establishingto
Idleoccurs when
EndEstablishthrows an exception.
- The transition from
Establishingto
Establishedoccurs when
EndEstablishreturns successfully.
- The transition from
Establishedto
Reestablishingoccurs when a registration refresh failure
occurs due to a connection drop (applies only to registered
application endpoints).
- The transition from
Reestablishingto
Establishingoccurs when the connection is restored and the
registration is refreshed (applies only to registered application
endpoints).
- The transition from
Establishedto
Terminatingoccurs when
BeginTerminateis called or when a forced deregistration by
the server occurs.
- The transition from
Establishedto
Terminatingoccurs when a registration refresh fails (applies
only to user endpoints).
- The transition from
Idleto
Terminatingoccurs when
BeginTerminateis called on the endpoint.
- The transition from
Reestablishingto
Terminatingoccurs when
BeginTerminateis called on the endpoint.
- The transition from
Terminatingto
Terminatedoccurs when
EndTerminatecompletes.
LocalEndpointSettings
Class
The LocalEndpointSettingsclass contains the settings needed to initialize a LocalEndpointobject.
LocalEndpointSettings Constructors
The LocalEndpointSettingsclass has the following constructors.
![]() |
|
---|---|
// Initializes a LocalEndpointSettings object. protected LocalEndpointSettings(string ownerUri); // Initializes a LocalEndpointSettings object. public LocalEndpointSettings(string ownerUri, string serverName, int serverPort); |
LocalEndpointSettings Properties
The following are the public properties on the LocalEndpointSettingsclass.
![]() |
|
---|---|
// Gets the URI of the owner of this endpoint. public string OwnerUri {get;} // Gets or sets whether to query for the provisioning data when the endpoint is established. // By default, the value is set to false and hence by default we will query for provisioning data on start up. public bool ProvisioningDataDisabled {get; set;} // Gets or sets the current state of the call screening feature. // If enabled, incoming calls are screened based on the user's state as perceived by the // remote participant. // The default value is false. public bool PresenceBasedScreeningDisabled {get; set;} // Gets or sets the methods supported by a registered endpoint. // By default, this is set to support subscription and signaling sessions. public string RegisterMethods {get; set;} // Gets or sets the list of content types that can be supported by the endpoint. // The content type of any MIME part with handling="required" should be in this list. // Otherwise, incoming calls will be rejected automatically. public IEnumerable<ContentType> SupportedMimePartContentTypes {get; set;} // Gets or sets the endpoint user agent string. // This string is used to build the overall UserAgent string for the endpoint. // This must be set only if each endpoint's user agent string is different. // If all endpoints in the application have the same user agent string, only set the application // user agent string in CollaborationPlatform. // The overall UserAgent string is formed by combining a default user agent string with // CollaborationPlatform.ApplicationUserAgent and LocalEndpoint.EndpointUserAgent public string EndpointUserAgent {get; set;} // Gets or sets whether the endpoint will try to publish quality metrics for the audio calls. // If set to true, the media quality metrics will not be published to the QoE server. Otherwise, by default, // the media quality metrics will be published. // The default value is false. Applications can override this default value. public bool PublishingQoeMetricsDisabled {get; set;} // Gets or sets the locality of the user. // If the application sets this value, it overrides the default // behavior of retrieving the value from the registration response. public bool? IsOutsideCorporateNetwork {get; set;} // Gets the type of user agent that this endpoint represents. public EndpointType EndpointType {get; } // Gets the subtype of user agent that this endpoint represents. public EndpointSubtype EndpointSubtype {get; } |
LocalEndpoint Constructors
There are no public contructors on the LocalEndpointclass. Application writers should use the classes derived from LocalEndpoint: ApplicationEndpointand UserEndpoint.
LocalEndpoint Properties
The following are the public properties on the LocalEndpointclass.
![]() |
|
---|---|
// Gets the locality of the user. // The default value (false) will be returned // if the application has not overridden the value in LocalEndpointSettings // and the value was not present in the registration response. public bool IsOutsideCorporateNetwork {get;} // Gets the type of user agent that this endpoint represents. public EndpointType EndpointType{get;} // Gets the subtype of the user agent that this endpoint represents. public EndpointSubType EndpointSubType{get;} // Gets the RealTimeEndpoint wrapped by this endpoint. public RealTimeEndpoint InnerEndpoint{get;} // Gets the platform that this endpoint is bound to. public CollaborationPlatform Platform{get;} // Gets the state of the local endpoint. public LocalEndpointState State{get;} // Gets the URI of the owner of the endpoint. public virtual string OwnerUri{get;} // Gets the phone URI of the owner of the endpoint. public abstract string OwnerPhoneUri{get;} // Gets the display name of the owner of the endpoint. public string OwnerDisplayName{get; protected set;} // Gets the URI of the endpoint. public string EndpointUri{get;} // Gets the default domain for the endpoint URI. public string DefaultDomain{get;} // Gets the phone context for the endpoint. // Phone context information is retrieved from provisioning data. // If user level value is available in provisioning data, then this property returns that value. // Otherwise, the pool level value is returned. // If provisioning data is not available, this property returns an empty string. public string PhoneContext{get;} // Gets the conference services object used to schedule conferences // and to perform other conference tasks. public ConferenceServices ConferenceServices{get;} // Gets the service that can be used to subscribe for presence information. // Supported only if the endpoint is registered on a server. public RemotePresence RemotePresence{get;} // Gets the service used to publish presence data for this endpoint. // Supported only if the endpoin tis registered on a server. public LocalOwnerPresence LocalOwnerPresence{get;} // Gets the current state of the call screening feature. // If enabled, incoming calls are screened based on the user's state as perceived by the // remote participant. public bool PresenceBasedScreeningDisabled {get;} // Gets the methods supported by a registered endpoint. // By default, this is set to support subscription and signaling sessions. public string RegisterMethods{get;} // Gets the list of content types that can be supported by the endpoint. // The content type of any mime part with handling=required should be in this list. // Otherwise, incoming calls will be rejected automatically. public IEnumerable<ContentType> SupportedMimePartContentTypes {get; } // Gets the user agent string that will be used for messages. // This is formed by combining a default user agent string with // CollaborationPlatform.ApplicationUserAgent and // LocalEndpoint.EndpointUserAgent. public string UserAgent {get;} // Gets the flag that controls whether the endpoint // will attempt to publish quality metrics for audio calls. public bool PublishingQoeMetricsDisabled {get;} // Gets whether provisioning data query has been disabled. public bool ProvisioningDataDisabled {get; set;} // Gets whether the provisioning data autorefresh has been disabled. public bool ProvisioningDataAutoRefreshDisabled {get;} |
The following table lists frequently-used properties of the LocalEndpointclass, and their descriptions.
Property | Description |
---|---|
EndpointType |
The type can be User, Application, Gateway, or Conference. User endpoints are always of type User. Application endpoints can be any of the four types. Setting the endpoint type translates to feature tag parameters in the Contactheader, with the following correspondences: Applicationtranslates to “automata”, Gatewaytranslates to “isGateway”, and Conferencetranslates to “isFocus”. |
EndpointSubtype |
This can be set only for application endpoints whose EndpointTypeproperty is set to Application. The EndpointSubtypeproperty corresponds to the “actor=” parameter in the Contactheader. A value of Nonemeans that the “actor =” parameter is missing from the Contactheader. The remaining values have the following correspondences: Principalcorresponds to ”principal”, MessageTakercorresponds to”msg-taker”, Attendantcorresponds to”attendant”, and Informationcorresponds to”information”. |
InnerEndpoint |
The RealTimeEndpointobject that is wrapped by the LocalEndpoint. This is needed only for advanced scenarios. |
Platform |
The CollaborationPlatformobject that corresponds to this endpoint. |
State |
The LocalEndpointstate, a value of the LocalEndpointStateenumeration. The possible values are Idle, Establishing, Established, Reestablishing, Terminating, and Terminated. For an explanation of the states and transitions between states, see the LocalEndpoint Events section in this topic. |
OwnerUri |
The URI with which the endpoint was created. |
OwnerPhoneUri |
The phone URI of the endpoint. This member is overridden in derived classes. |
OwnerDisplayName |
The display name of the endpoint. This member is overridden in derived classes. |
EndpointUri |
For registered endpoints this is the dynamic Globally Routable UserAgent URI (GRUU) returned by Office Communications Server during registration. For unregistered application endpoints this will contain the value of the ActiveGruuproperty on the platform after the endpoint is established. The GRUU is set on any message that the endpoint sends as the Contactheader. Application endpoints use the platform’s ActiveGruuproperty as the Contactheader for privileged operations even if it has a dynamic GRUU. |
DefaultDomain |
The default domain of the endpoint, as derived from the Uriproperty on the endpoint. |
PhoneContext |
The phone context to be used for telURIs. This is received through inband data. |
ConferenceServices |
This member is used to perform several conference-related tasks for the endpoint. |
RemotePresence |
This service is used to subscribe to presence information. Applicable only to registered endpoints. |
LocalOwnerPresence |
This service is used to publish presence information. Applicable only to registered endpoints. |
PresenceBasedScreeningEnabled |
If enabled, incoming calls are screened based on the user's state as perceived by the remote participant. Applicable only to registered endpoints. |
RegisterMethods |
Gets or sets the methods supported by a registered endpoint. This property should usually be set before the endpoint’s Establishmethod is called. If set later it will take effect when registration refresh occurs. The default value should be sufficient for most applications: "\"Service,Notify,Benotify,Message,Info,Options,Invite\"". |
SupportedMimePartContentTypes |
Specifies the content types that the application supports. The content type of any mime part with handling=required should be in this list. Otherwise, incoming calls will be rejected automatically. |
LocalEndpoint Methods
The following are the public methods on the LocalEndpointclass.
![]() |
|
---|---|
// Returns a snapshot of the conversations managed by this endpoint. public ReadOnlyCollection<Conversation> GetConversations(); // Initializes the endpoint so that it is ready for receiving incoming messages. public IAsyncResult BeginEstablish(AsyncCallback userCallback, object state); // Initializes the endpoint so that it is ready for receiving incoming messages. public IAsyncResult BeginEstablish(IEnumerable<SignalingHeader> additionalHeaders, AsyncCallback userCallback, object state); // Completes the establish operation. public SipResponseData EndEstablish(IAsyncResult result); //Terminates the endpoint and cleans up active sessions and resources. //The endpoint is no longer usable. public IAsyncResult BeginTerminate(AsyncCallback userCallback, object state); // Completes the asynchronous Terminate operation. public void EndTerminate(IAsyncResult result) // Initiates an operation to query for the latest provisioning data. public IAsyncResult BeginGetProvisioningData(AsyncCallback userCallback, object state); // Completes the operation to query for the latest provisioning data public ProvisioningData EndGetProvisioningData(IAsyncResult result); // Adds an application handler for handling incoming calls of a specific type. // Only one delegate can be registered for each TCall type. // Subsequent calls to this method will overwrite the previously set value. public void RegisterForIncomingCall<TCall>(IncomingCallDelegate<TCall> incomingCallDelegate) where TCall : Call; // Removes a previously added handler that was handling incoming calls of a specific type. public void UnRegisterForIncomingCall<TCall>(IncomingCallDelegate<TCall> incomingCallDelegate) where TCall:Call; // Handles an incoming signaling session. // A derived class can override this method to process an incoming SignalingSession before UCMA 2.0 Core processes it. protected virtual bool HandleSignalingSession(SessionReceivedEventArgs sessionReceivedEventArgs); |
The following table lists commonly-used methods of the LocalEndpointclass, and their descriptions.
Method | Description |
---|---|
GetConversations |
Gets a snapshot of the conversations managed by this endpoint. |
BeginGetProvisioningData |
Returns the cached inband provisioning data, or retrieves it from the server if not already present. |
BeginEstablish |
Initializes the endpoint so that it is ready to receive incoming messages. After calling this method, call EndEstablishto complete the operation. Among other things, this causes endpoints to be registered using the appropriate type. This method can be called only after CollaborationPlatform.Startuphas been called successfully. |
BeginTerminate |
Terminates the endpoint and cleans up active sessions and resources. After calling this method, call EndTerminateto complete the operation. CollaborationPlatform.Shutdownautomatically terminates the endpoints as well. After Terminateis called, the endpoint is unusable. |
RegisterForIncomingCall< |
Adds an application handler to handle incoming calls of a specific type. Only one delegate can be registered for each type. Subsequent calls to this method overwrite the previously set value. |
UnregisterForIncomingCall< |
Removes a previously added handler that handled incoming calls of a specific type. |
LocalEndpoint Events
The following table lists frequently-used events on the LocalEndpointclass, and their descriptions.
Event | Description |
---|---|
StateChanged |
Raised when the state of the endpoint changes. The StateChangedevent is raised according to the state transitions in the illustrations that follow this table. The first illustration shows the state transitions for user endpoints and unregistered application endpoints. The second illustration shows the state transitions for registered application endpoints. |
RepublishingRequired |
Raised when the Registerresponse contains a "presence-state" header with Register-action, which requires the application to republish some of the data. If the Register-actionheader value is "Added”, applications are expected to publish all categories ( eventArgwill have PartialRepublishset to false). If the Register-actionheader value is "fixed”, selected category data might need to be republished ( PartialRepublishwill be set to true). If the Register-actionheader value is “Refreshed”, the event is not raised, as nothing needs to be republished. In case of “fixed” or “added”, Unified Communications Managed API 2.0 Core SDK also refreshes all current subscriptions before raising this event. |
ConferenceInvitationReceived |
Notifies an application when a conference invitation is received. |